Hello hello! This is a the first part of a three part series were we will be exploring various web technologies by supercharging a simple HTML5 website. We will cover from creating a build process to deploying using GitHub Actions (second part) and other providers like AWS amplify.
In this first part tutorial we will be adding NPM to a standalone HTML5 site, adding a local server with an awesome handy NPM package with live refresh so it auto reloads with any code changes and adding a build process (totally optional but is fun to only have 1 html file with one css and js minified.
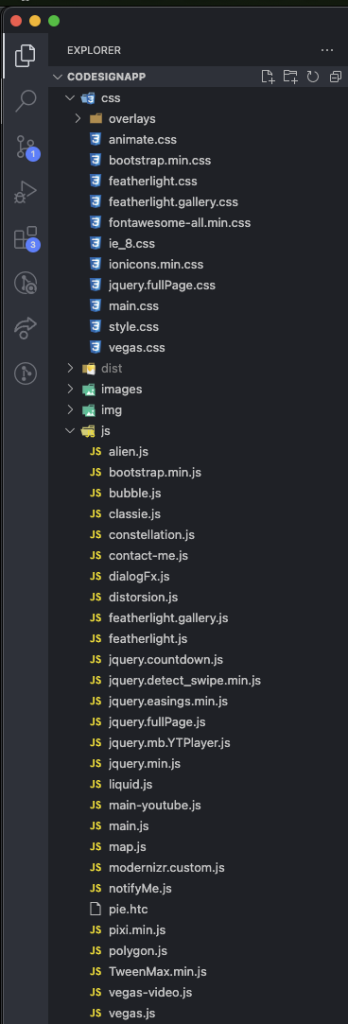
So this is the project I’m going to be working on, as you can see it’s just standard HTML with CSS and JavaScript with good oldies like JQuery and other libraries.
Step 1: Setting up NPM: We’ll start by initializing NPM in our project. Open your terminal and navigate to your project’s root directory. Run the following command to create a package.json file with default settings:
npm init -y
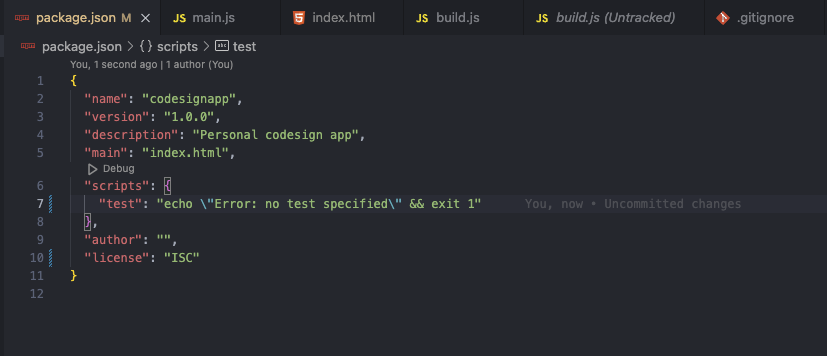
This will create a package.json with default values, feel free to change the name, version and description to match your project. Also keep in mind to change the entry point to index.html instead of index.js since this is an HTML5 APP
Step 2: Install a local server package Next, you’ll need a package to run a local server for testing. One popular choice is live-server
. Install it by running the following command:
npm install live-server --save-dev
This will install live-server
as a development dependency and save it in the package.json
file.
This creates a script named “start” that will run the live-server
package.

To start the local server In your terminal or command prompt, run the following command to start the local server:
npm start
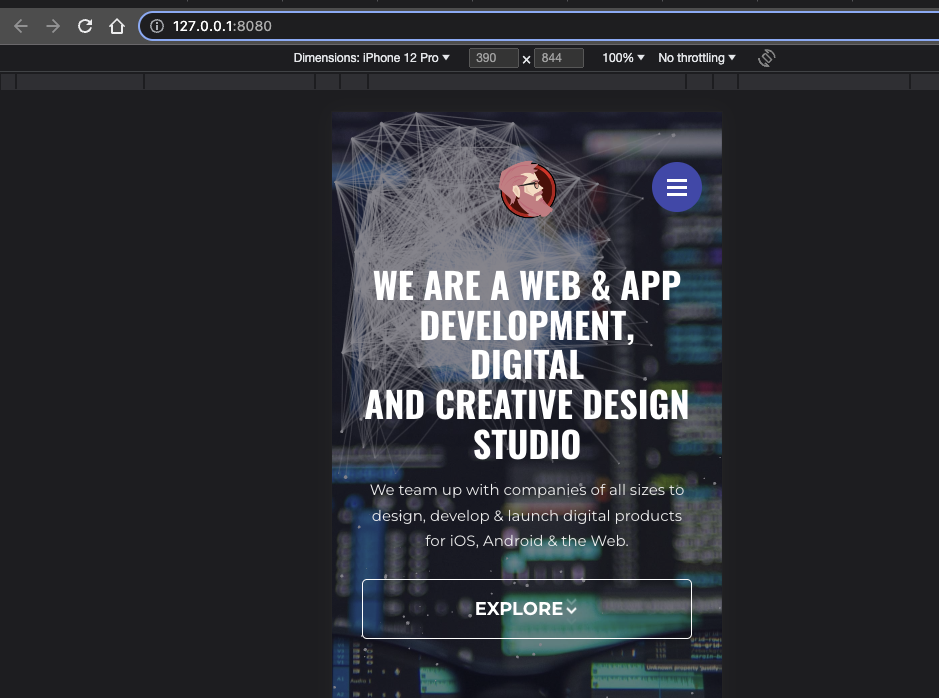
This will create a local server and start your site! And it listen for changes on the code and automatically reload it. Very simple and useful

Step 3: Lets minimize! Installing Required Packages:
Next up we will be installing this two awesome packages:
UglifyJS is a JavaScript minification tool that helps optimize your JavaScript code by reducing its size and removing unnecessary characters like whitespace, comments, and unused code. It also performs various code transformations to improve performance and reduce the overall file size. Minifying your JavaScript files with UglifyJS can lead to faster loading times and improved website performance.
Rimraf is a command-line utility used for deleting files and directories. It provides a convenient way to clean up your build directory or any other directories/files you want to remove. Rimraf ensures that the deletion is thorough and recursive, handling files and directories with different permissions, and it works across different platforms.
To utilize UglifyJS and Rimraf, we need to install them as development dependencies. Run the following command to install them:
npm install uglify-js rimraf --save-dev
Step 4: Configuring Build Script: Open your package.json file and add the following script section:
"scripts": {
"build": "npm run build:js && npm run build:css && npm run edit:index",
"build:js": "uglifyjs js/*.js -o dist/minified.js",
"build:css": "cleancss css/*.css -o dist/minified.css", "edit:index": "node build-scripts/edit-index.js",
"clean": "rimraf dist"
}
This scripts will get all css and js files, minify them and copy the result to the dist folder.
Step 5: Creating the Edit Index Script: Create a new file named edit-index.js
inside a build-scripts
directory. Add the following code to the file:
const fs = require('fs');
const cheerio = require('cheerio');
const indexPath = 'index.html';
const cssPath = 'minified.css';
const jsPath = 'minified.js';
const distIndexPath = 'dist/index.html';
fs.readFile(indexPath, 'utf8', (err, data) => {
if (err) {
console.error('Error reading index.html:', err);
return;
}
const $ = cheerio.load(data);
// Remove existing CSS includes
$('link[rel="stylesheet"]').remove();
// Remove existing JS includes
$('script[src]').remove();
// Add minified CSS
$('<link rel="stylesheet" href="' + cssPath + '">').appendTo('head');
// Add minified JS
$('<script src="' + jsPath + '"></script>').appendTo('body');
// Save modified HTML to dist folder
fs.writeFile(distIndexPath, $.html(), (err) => {
if (err) {
console.error('Error writing modified index.html:', err);
return;
}
console.log('Modified index.html successfully saved in dist folder');
});
});
This code snippet is used to modify an HTML file (index.html) by removing existing CSS and JavaScript includes and adding references to minified CSS and JavaScript files. It utilizes the fs
module to read and write files, and the cheerio
library to parse and manipulate the HTML document.
Step 6: Add copy images step: We will be adding a new script: build:images, which uses the cp command to copy the images from your source directory to the output directory
"build:images": "cp -R images/ dist/images/"
Our final package.json will look like this:
"scripts": {
"build": "npm run build:js && npm run build:css && npm run edit:index && npm run build:images",
"build:js": "uglifyjs js/*.js -o dist/minified.js",
"build:css": "cleancss css/*.css -o dist/minified.css",
"build:images": "cp -R images/ dist/images/",
"edit:index": "node build-scripts/edit-index.js",
"clean": "rimraf dist"
}
Step 7: Building the Project: Now, we can run the build command to generate the minified and optimized files. Open your terminal and run the following command:
npm run build
And that’s it! You should have 1 html, css and js file with the images copied on your dist folder!
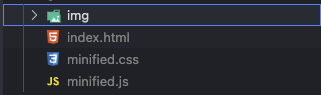
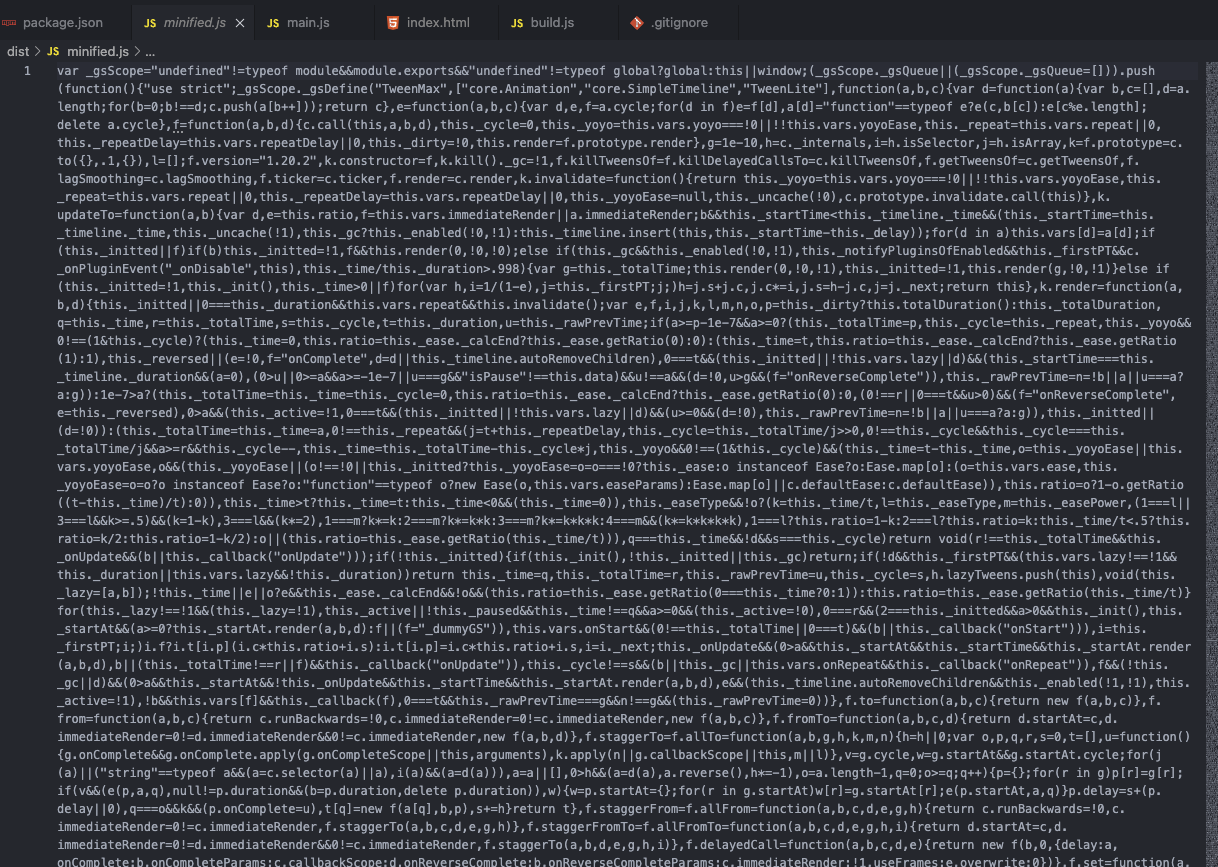
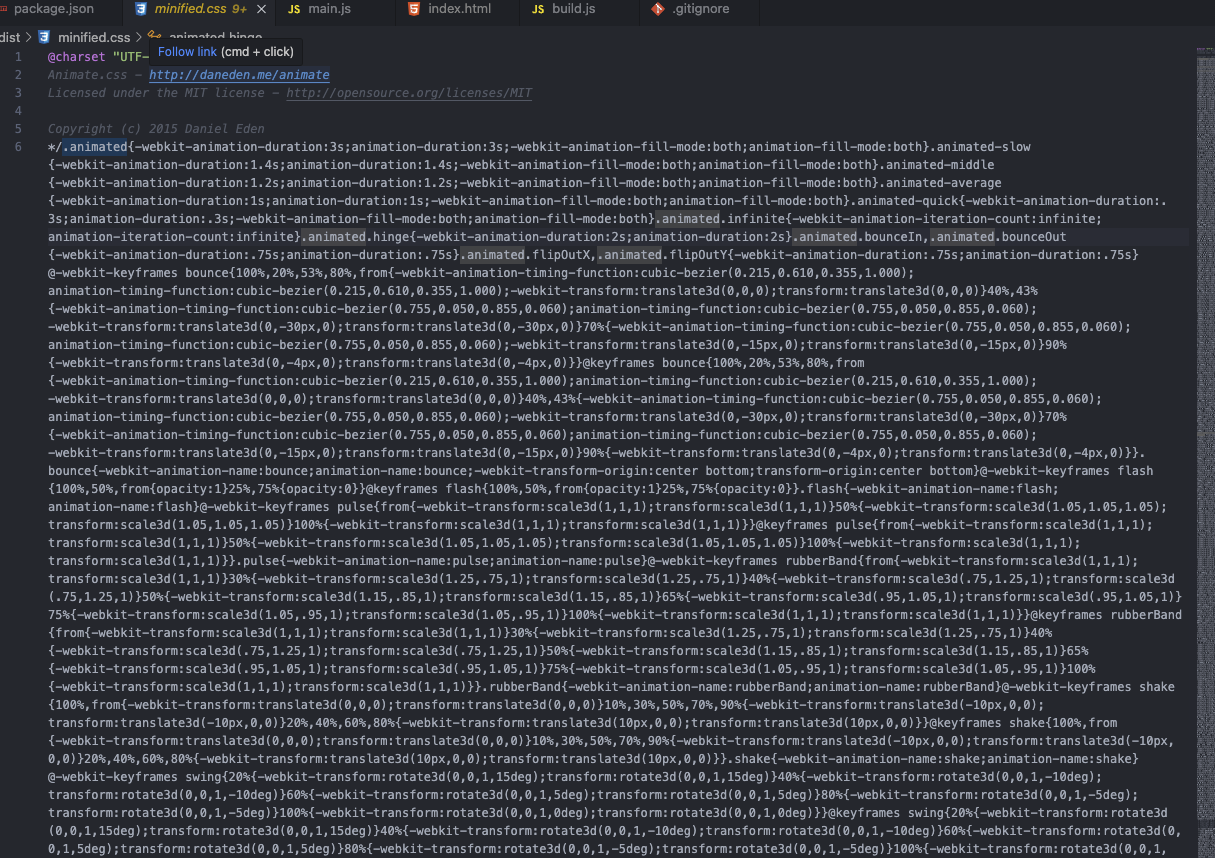
Step 8: Cleaning the Dist Folder: To ensure a clean build, we can add a step to delete the contents of the dist
folder before each build. Add the following script to your package.json file:
scripts": {
...
"clean": "rimraf dist"
}
Conclusion: By leveraging NPM, UglifyJS, and Rimraf, we’ve streamlined our front-end build process. We automated the minification and bundling of JavaScript and CSS files, edited the index.html file to include the minified files, and cleaned the build directory for a fresh start. Implementing these steps will improve your development workflow and optimize your website’s performance.