So I just found myself on creating a video header background for a client’s site, and needed to join about 7 videos, compress them to an aceptable size for the web, and didn’t really want to download iMovie or use a video editing software. My approach was to use a simple script. Whether you’re working on a Mac or another Unix-like system, this should work for you. Let’s dive in!
Why Would You Want to Do This?
Sometimes you’ve got a bunch of video clips from your phone, camera, or other sources, and you want to make them into one seamless video. Maybe it’s for a vlog, a presentation, or just to share with friends. But those raw video files can be huge, and stitching them together can be a pain if you don’t know the right tools. That’s where this script comes in handy!
What You’ll Need
- A Mac or Unix-like system: This script uses
ffmpeg
, a powerful and free tool for handling videos. - Some basic terminal knowledge: Don’t worry, I’ll walk you through it!
The Script
Here’s the magic script that does it all. Just copy this into a text file, save it as something like compress_and_join.sh
, and make sure it’s executable.
#!/bin/bash
# Ensure we're working in the directory where the script is located
cd "$(dirname "$0")"
# Step 1: Compress each video and create intermediate files for concatenation
index=0
for f in *.mov; do
if [ -f "$f" ]; then
index=$((index + 1))
output="compressed_${index}.mp4"
# Compress the video
ffmpeg -i "$f" -vcodec libx264 -crf 28 -preset fast -vf "scale=-2:720" -an "$output"
fi
done
# Step 2: Generate a list of all compressed video files
concat_list="concat_list.txt"
rm -f $concat_list
for f in compressed_*.mp4; do
if [ -f "$f" ]; then
echo "file '$f'" >> $concat_list
fi
done
# Step 3: Concatenate all videos by re-encoding them into a single stream
ffmpeg -f concat -safe 0 -i $concat_list -c:v libx264 -crf 28 -preset fast -c:a copy final_output.mp4
# Cleanup temporary files
rm $concat_list
rm compressed_*.mp4
How to Use the Script
- Save Your Script: Drop this script into the folder where your
.mov
video files are located. - Make It Executable: Open your Terminal and navigate to the folder with the script. Run the command:
chmod +x compress_and_join.sh
Run It: Now, just run the script:
./compress_and_join.sh
And that’s it! The script will do all the heavy lifting—compressing each video and then joining them into a single file called final_output.mp4
.
Why This Works Like a Charm
- Compression: The script uses
ffmpeg
to compress each video so they take up less space but still look good. - Re-encoding: Instead of just slapping the videos together (which can cause issues), this script re-encodes them into a single smooth video.
- Simple: You don’t need any special software or hours of fiddling around—just run the script and let it do its thing.
Wrapping Up
If you’re a video enthusiast or just someone who occasionally needs to work with video files, this script is a real time-saver. It’s a simple, effective way to compress and join videos without needing to mess with complex editing software. Plus, once you’ve set it up, you can reuse it whenever you need to—just drop it in a folder with your videos, and you’re good to go.
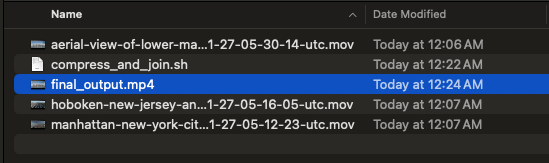